Cost/Wear: Part 1

Journal 06
I’m really keen to get in the basic functionality that I’ve been thinking about this entire time: Cost per wear (CpW).
To start, I need to update my database to have a cost column. It will then get even trickier to figure out the best way to capture the number of wears. But we’ll get to that soon.
Showing Cost
First step is to add this to the database.
I need to generate a migration first:
$ bin/rails generate migration AddPriceToArticle cost:decimal
Which is then migrated, committed to Github and pushed to Heroku. Ready for any changes I make that might need that database column.
Once this was done; I added it to the Articles Controller as a permitted param then went about adding it in to my form so I can add values for it. Here’s the code to bring it in:
<div class="form-field__input">
<div class="cost-input-wrapper">
<span class="cost-input-wrapper__symbol">$</span>
<%= form.text_field :cost, class: "input-text", placeholder: "00.00", value: display_cost(@article.cost) %>
</div>
</div>
The idea is to have the $ sign sitting outside of the text field; and for the text field to nicely maintain its full width amount, so it lines up with the rest of the boxes.
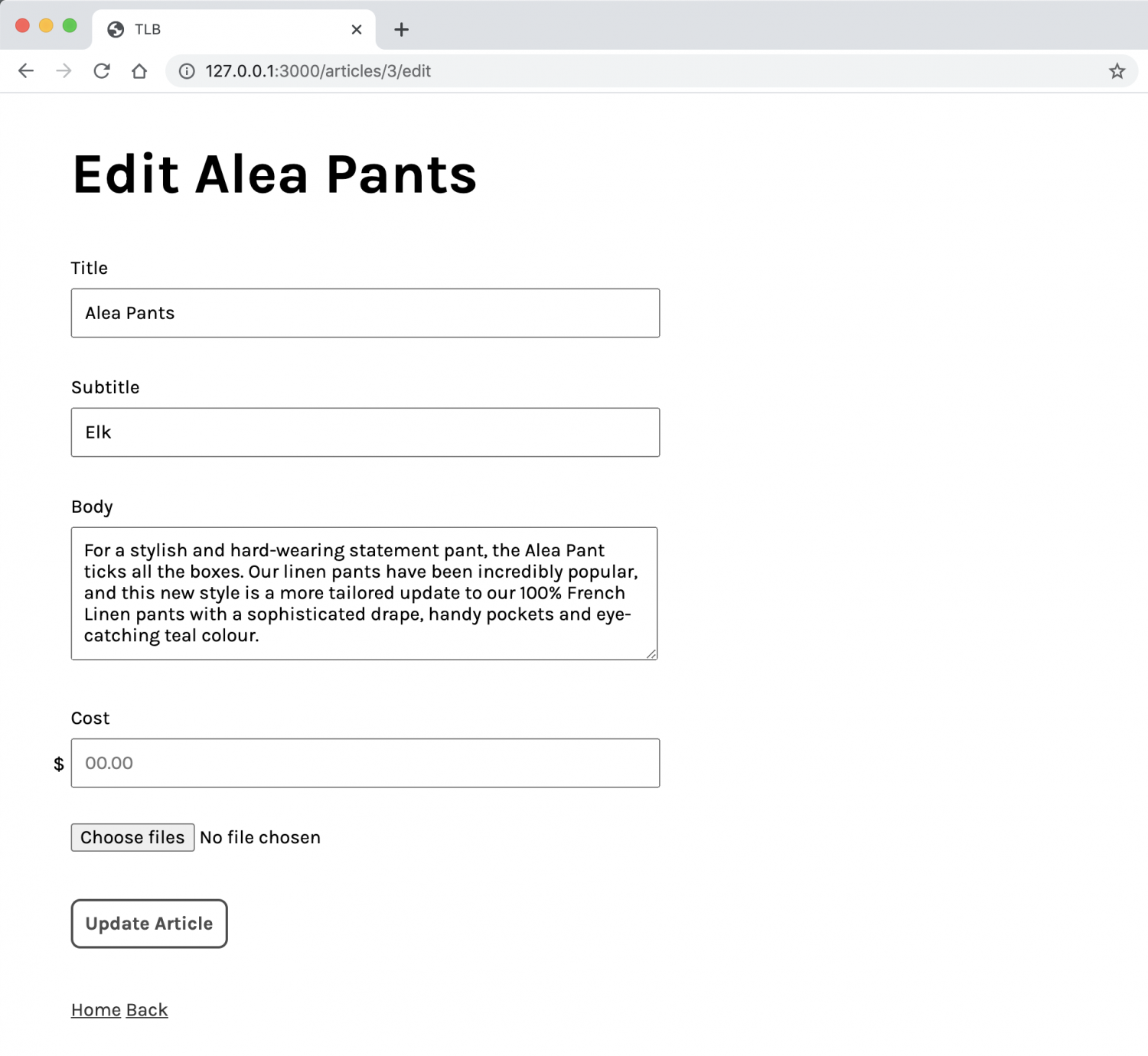
Also note the placeholder to give a handy hint about the format to enter the cost in.
Finally there is a value calling the display_cost Helper I’ve created:
def display_cost(cost)
if cost
"%.2f" % cost
else
""
end
end
This ensures the value is given to two decimals. (It was shortening it previously. $35.10 was becoming $35.1 which just doesn’t look like money).
Also brought in the if / else behaviour to only show the cost amount when it exists (and not otherwise break the page).
Pretty happy with how this is turning out so far – there’s a fair bit more to do to get the CpW up and running though!